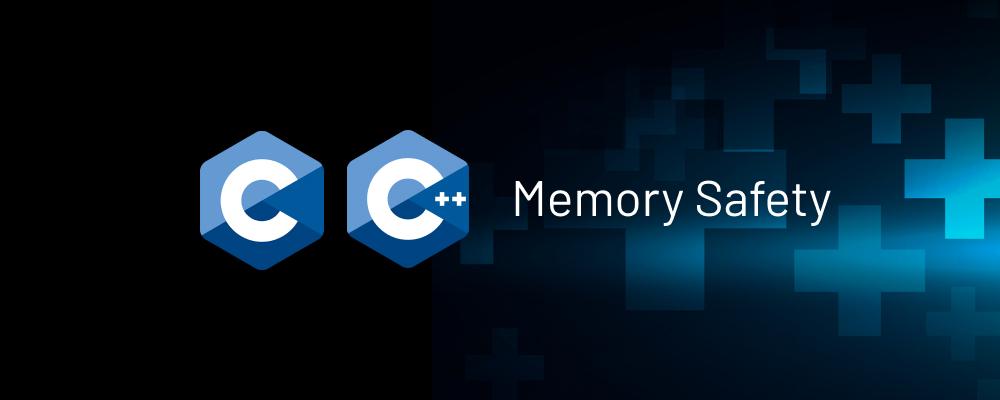
Recent Initiatives to Improve C and C++ Memory Safety
Memory safety is crucial in programming languages because it helps prevent various dangerous and unintended behaviors that can lead to software vulnerabilities, crashes, and security breaches. Memory safety ensures that a program only accesses valid memory locations, handles data correctly, and avoids corrupting the memory space allocated to other program parts.
While C and C++ offer high performance and fine-grained control over system resources, these features come at the cost of memory safety. The lack of built-in memory safety mechanisms (like garbage collection, bounds checking, or automatic reference counting) means that memory-related bugs are easier to introduce and harder to track down, posing risks for both reliability and security.
Several initiatives are underway to make C and C++ memory-safe languages, addressing key concerns like buffer overflows, null pointer dereferencing, and other common memory-related vulnerabilities. Some of the most notable efforts include:
1. Rust as an Alternative
Rust is often seen as a modern alternative to C and C++ due to its emphasis on memory safety without sacrificing performance. While it's a separate language, it has been explicitly designed to address many problems that C and C++ struggle with. It enforces ownership and borrowing rules at compile-time, ensuring that issues like dangling pointers, data races, and buffer overflows are prevented.
The Linux kernel, traditionally written in C, now has support for writing device drivers in Rust. At the end of 2024, it was estimated that over 15% of new kernel modules were being developed in Rust.
2. Safe C and C++ Proposals
Various projects aim to make C and C++ safer while maintaining compatibility with existing codebases.
- Safe C++: Some C++ language proposals include mechanisms to improve memory safety by using modern C++ features and enforcing better memory management practices.
- C++ Core Guidelines: The C++ Core Guidelines, developed by experts like Bjarne Stroustrup, emphasize safer coding practices to avoid undefined behaviors and memory issues. For instance, it recommends guidelines for avoiding raw pointers and encourages the use of smart pointers instead.
3. Memory Safety Tools for C and C++
Several tools and libraries exist to catch memory errors at compile-time or runtime.
- Sanitizers: Tools like AddressSanitizer (ASan), MemorySanitizer (MSan), and ThreadSanitizer help detect various memory-related bugs during development by adding runtime checks. These tools can catch issues such as memory leaks, use-after-free, and out-of-bounds access.
- Valgrind: This tool is widely used to detect memory leaks, memory corruption, and undefined memory usage in C and C++ applications. It helps detect and debug memory issues that might not be immediately apparent during normal operation.
- Clang's Safe Language Extension (Safe C++): Clang's safe language extension includes tools that provide extra checks for memory safety, attempting to catch errors like buffer overflows and improper use of pointers.
4. Safe Memory Allocation Techniques
Some efforts are focused on improving how memory is allocated and managed in C and C++. These include libraries and tools designed to enhance memory safety.
- The C++ Standard Library: Newer versions of the C++ Standard Library are incorporating safer memory management techniques, such as the use of std::shared_ptr and std::unique_ptr, which help avoid common memory management mistakes.
- Memory Safe Allocators: Some developers are building "safe" allocators and containers that provide better memory safety guarantees, using techniques like bounds checking and automatic deallocation to reduce risks.
- Scoped Allocators: Scoped allocators are used to ensure that memory is properly managed within a defined scope, preventing memory leaks and dangling pointers.
5. Formal Verification and Static Analysis
There are ongoing efforts to apply formal verification to C and C++ programs to ensure their correctness and memory safety. Tools like Frama-C and CBMC (C Bounded Model Checker) use formal methods to detect potential errors like buffer overflows or null pointer dereferencing before runtime.
Static analysis tools, such as the Clang Static Analyzer and Coverity, analyze code without executing it, detecting memory management problems like uninitialized memory, buffer overruns, and memory leaks.
6. Memory-Safe C Compilers
Some compilers are being modified or extended to promote safer memory management practices for C.
- CCured: CCured is an extension of the C language designed to provide automatic memory safety by tracking pointer types and checking pointer usage at compile-time. It tries to catch common C vulnerabilities such as dangling pointers and buffer overflows.
- SafeC: A modified version of the C language, SafeC is aimed at eliminating unsafe memory manipulations in C, ensuring better memory safety at compile-time.
- GCC and Clang with Memory Safety Options: Modern GCC and Clang compilers have been enhanced to provide memory safety features such as bounds checking and the detection of uninitialized memory accesses.
7. Automatic Memory Management Enhancements
Another direction is to introduce elements of automatic memory management, such as garbage collection, into C and C++. While this is not a typical feature in these languages, certain efforts are exploring this possibility.
There are experimental projects exploring how automatic garbage collection could be integrated into C++ without sacrificing performance. However, C++ does not yet have native garbage collection, and developers must rely on techniques like RAII (Resource Acquisition Is Initialization) and smart pointers.
8. C++ Memory Safety with Safe Data Structures
Another line of development involves creating safer data structures in C++ that reduce memory safety risks. For example, new data structures in libraries like Abseil (from Google) or Folly (from Facebook) aim to be more resilient to memory safety issues. These data structures incorporate features that help ensure safe memory use.
Conclusion
While C and C++ are inherently low-level languages, meaning they provide fine-grained control over memory, this also makes them prone to dangerous memory errors. Efforts to make them more memory-safe involve adopting new programming practices, designing safer language extensions, creating new tooling (such as sanitizers and static analysis tools), and even exploring formal verification and safe memory management techniques. While no one approach can fully solve all the challenges, the collective aim is to reduce the likelihood of memory-related bugs and make these powerful languages safer to use.
References
- Rust for Linux: https://en.wikipedia.org/wiki/Rust_for_Linux
- TrapC: https://vimeo.com/1028578347
- Fil-C: https://github.com/pizlonator/llvm-project-deluge/blob/deluge/Manifesto.md
- Safe C++: https://safecpp.org/P3390R0.html
- C++ Core Guidelines: https://isocpp.github.io/CppCoreGuidelines/CppCoreGuidelines