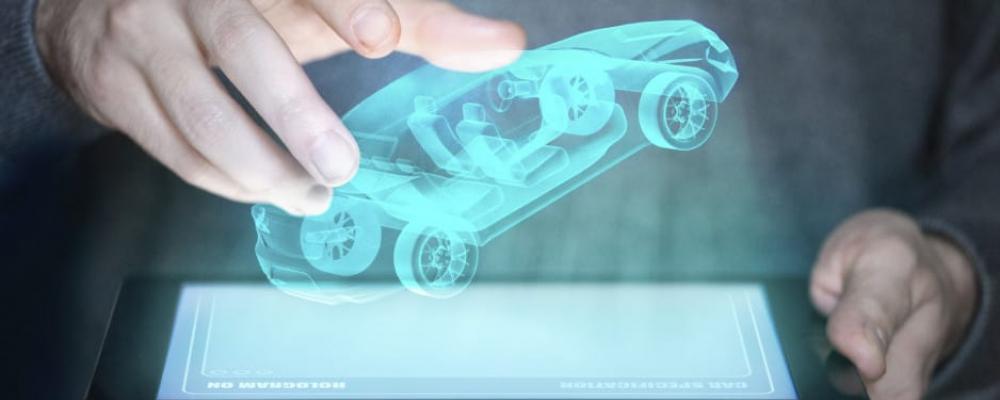
An Introduction to Qt3D
In this blog post, we'll take a brief look at one of the newest Qt modules, Qt3D, which was introduced in Qt 5.5.0.
What is Qt3D?
Qt3D is a new Qt module that provides support for 2D and 3D rendering. It also provides a generic framework for supporting simulations that go beyond just rendering, and can include features like physics, audio, collision detection, artificial intelligence and path finding.
It has APIs for both C++ and Qt Quick (QML) and uses OpenGL as the underlying technology.
Current Status
Qt3D has been in development for some time, and its scope has expanded, in part because OpenGL has been evolving. Because of the many changes over time, the current version is indicated as version 2.0.
Qt3D was first released as part of Qt 5.5.0, where it is considered to be a technology preview, meaning that some of the APIs are still subject to change. It is expected to be API stable in the Qt 5.6.0 release, coming at the end of 2015.
Which Platforms are Supported?
In the Qt 5.5.0 release, Qt3D is supported on Microsoft Windows, desktop and embedded Linux, Mac OS X and Android platforms. The Mac iOS and WinRT platforms are not yet supported. Additional platforms should be offered in Qt 5.6.0.
How Much OpenGL Do I Need to Know? How Much 3D Do I Need to Know?
Qt3D hides many of the details of OpenGL and its APIs from developers when using it, but depending on what features you use, you should have an understanding of 3D concepts such as:
- meshes
- materials
- shaders
- shadow mapping
- ambient occlusion
- high dynamic range
- deferred rendering
- multitexturing
- instanced rendering
- uniform buffer objects
If you only need basic 3D functionality, Qt3D may be overly complex and you might want to look at using the Qt Canvas 3D module instead.
What Exactly Does It Provide?
Qt3D provides a number of C++ classes in three modules. It also provides a number of QML elements, also divided into three modules.
QML Types
The QML types are organized into three modules: Qt3D, Qt3D.Input and Qt3D.Renderer.
The Qt3D QML module provides the core Qt3D QML types. QML programs gain access to the elements using the QML statement:
import Qt3D 2.0
The provided QML elements are the following:
- Camera
- CameraLens
- Component3D
- Configuration
- Entity
- EntityLoader
- MatrixTransform
- Node
- NodeInstantiator
The Qt3D.Input module provides QML types related to Qt3D user input and is accessed with the directive:
import Qt3D.Input 2.0
The elements are:
- KeyEvent
- KeyboardController
- KeyboardInput
The third module is Qt3D.Renderer, providing Qt3D QML types for rendering and is imported using:
import Qt3D.Renderer 2.0
The associated QML elements are:
- AbstractTextureImage
- Annotation
- BlendState
- BlendStateSeparate
- FrameGraph
- FrameGraphNode
- Layer
- LayerFilter
- OpenGLFilter
- ParameterMapping
- PointLight
- SpotLight
- TextureImage
All of these elements are documented in the official Qt documentation.
C++ Classes
The C++ API is divided into three modules, in a similar manner to the QML modules: Qt3DCore, Qt3DInput and Qt3DRenderer.
To use the modules from a qmake project, you need to add these module names to your project file:
QT += 3dcore 3drenderer 3dinput
A Qt Quick (QML) application requires these additional modules:
QT += qml quick 3dquick
Qt3DCore
The Qt3DCore module provides the following C++ classes (all defined in the Qt3D namespace):
- ArrayAllocatingPolicy
- ListAllocatingPolicy
- ObjectLevelLockingPolicy
- QAbstractAspect
- QChangeArbiter
- QCircularBuffer
- QComponent
- QEntity
- QFrameAllocator
- QNode
- QOpenGLInformationService
- QResourceManager
- QServiceLocator
- QSystemInformationService
Qt3DInput
The Qt3DInput module provides the following C++ classes:
- Q3DKeyEvent
- QInputAspect
- QKeyboardController
- QKeyboardInput
Qt3DRenderer
The Qt3DRenderer module provides a large number of C++ classes, which are not currently documented, but will be once Qt3D is no longer at a tech preview status.
A Simple Example
The following simple program is provided as part of Qt3D as the "cylinder-qml" example. The source code for the QML portion is listed below.
(the following sample code is used with permission: Copyright © 2014 Klaralvdalens Datakonsult AB (KDAB)) import Qt3D 2.0 import Qt3D.Renderer 2.0 Entity { id: sceneRoot Camera { id: camera projectionType: CameraLens.PerspectiveProjection fieldOfView: 45 aspectRatio: 16/9 nearPlane: 0.1 farPlane: 1000.0 position: Qt.vector3d(0.0, 0.0, -20.0) upVector: Qt.vector3d(0.0, 1.0, 0.0) viewCenter: Qt.vector3d(0.0, 0.0, 0.0) } Configuration { controlledCamera: camera } FrameGraph { id: external_forward_renderer activeFrameGraph: ForwardRenderer { camera: camera clearColor: "black" } } components: [external_forward_renderer] CylinderMesh { id: mesh radius: 1 length: 3 rings: 100 slices: 20 } Transform { id: transform Scale { scale3D: Qt.vector3d(1.5, 1.5, 1.5) } Rotate { angle: 45 axis: Qt.vector3d(1, 0, 0) } } Material { id: material effect: Effect { } } Entity { id: mainEntity objectName: "mainEntity" components: [ mesh, material, transform ] } }
The example program illustrates some Qt3D QML elements including a top level Entity, Camera and FrameGraph. The cylinder is implemented using the CylinderMesh element. A screen shot is shown below.
In order for the example to run, there is some additional C++ code needed, which can be seen in the file main.cpp. Note that, typically, Qt3D programs will not run under the qmlscene program as some additional code is needed.
Approximately 30 examples 3 come with Qt3D. I encourage you to review and run them to gain more insight into the features of Qt3D.
Summary
Qt3D is a new module in Qt 5.5.0, considered to be at a tech preview state after a lengthy period of development. The Qt3D module should find wide applicability in areas such as 3D visualization, games and simulations, as well as allowing developers to implement features much more quickly and easily than when directly using the underlying OpenGL APIs.
The current version has a few rough edges, including incomplete documentation; this should be addressed in Qt 5.6.0 and future releases.
I hope to cover more details of Qt3D in a future blog post.
References
- Qt3D Module, Qt Documentation website, http://doc.qt.io/qt-5/qt3d-index.html
- Qt3D Overview, Qt Documentation website, http://doc.qt.io/qt-5/qt3d-overview.html
- Qt3D Examples, Qt Documentation website, http://doc.qt.io/qt-5/qt3d-examples.html